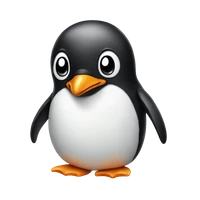
Shuu12121/CodeModernBERT-Owl
Sentence Similarity
•
Updated
•
41
•
2
code
stringlengths 11
173k
| docstring
stringlengths 2
593k
| func_name
stringlengths 2
189
| language
stringclasses 1
value | repo
stringclasses 844
values | path
stringlengths 11
294
| url
stringlengths 60
339
| license
stringclasses 4
values |
---|---|---|---|---|---|---|---|
public final CountedCompleter<?> firstComplete() {
for (int c;;) {
if ((c = pending) == 0)
return this;
else if (weakCompareAndSetPendingCount(c, c - 1))
return null;
}
} |
If this task's pending count is zero, returns this task;
otherwise decrements its pending count and returns {@code null}.
This method is designed to be used with {@link #nextComplete} in
completion traversal loops.
@return this task, if pending count was zero, else {@code null}
| CountedCompleter::firstComplete | java | Reginer/aosp-android-jar | android-34/src/java/util/concurrent/CountedCompleter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/java/util/concurrent/CountedCompleter.java | MIT |
public final CountedCompleter<?> nextComplete() {
CountedCompleter<?> p;
if ((p = completer) != null)
return p.firstComplete();
else {
quietlyComplete();
return null;
}
} |
If this task does not have a completer, invokes {@link
ForkJoinTask#quietlyComplete} and returns {@code null}. Or, if
the completer's pending count is non-zero, decrements that
pending count and returns {@code null}. Otherwise, returns the
completer. This method can be used as part of a completion
traversal loop for homogeneous task hierarchies:
<pre> {@code
for (CountedCompleter<?> c = firstComplete();
c != null;
c = c.nextComplete()) {
// ... process c ...
}}</pre>
@return the completer, or {@code null} if none
| CountedCompleter::nextComplete | java | Reginer/aosp-android-jar | android-34/src/java/util/concurrent/CountedCompleter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/java/util/concurrent/CountedCompleter.java | MIT |
public final void quietlyCompleteRoot() {
for (CountedCompleter<?> a = this, p;;) {
if ((p = a.completer) == null) {
a.quietlyComplete();
return;
}
a = p;
}
} |
Equivalent to {@code getRoot().quietlyComplete()}.
| CountedCompleter::quietlyCompleteRoot | java | Reginer/aosp-android-jar | android-34/src/java/util/concurrent/CountedCompleter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/java/util/concurrent/CountedCompleter.java | MIT |
public final void helpComplete(int maxTasks) {
ForkJoinPool.WorkQueue q; Thread t; boolean owned;
if (owned = (t = Thread.currentThread()) instanceof ForkJoinWorkerThread)
q = ((ForkJoinWorkerThread)t).workQueue;
else
q = ForkJoinPool.commonQueue();
if (q != null && maxTasks > 0)
q.helpComplete(this, owned, maxTasks);
} |
If this task has not completed, attempts to process at most the
given number of other unprocessed tasks for which this task is
on the completion path, if any are known to exist.
@param maxTasks the maximum number of tasks to process. If
less than or equal to zero, then no tasks are
processed.
| CountedCompleter::helpComplete | java | Reginer/aosp-android-jar | android-34/src/java/util/concurrent/CountedCompleter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/java/util/concurrent/CountedCompleter.java | MIT |
private static void dumpHprof() {
// hprof dumps are rather large, so ensure we don't fill the disk by generating
// hundreds of these that will live forever.
TreeSet<File> existingTombstones = new TreeSet<>();
for (File file : HEAP_DUMP_PATH.listFiles()) {
if (!file.isFile()) {
continue;
}
if (!file.getName().startsWith("fdtrack-")) {
continue;
}
existingTombstones.add(file);
}
if (existingTombstones.size() >= MAX_HEAP_DUMPS) {
for (int i = 0; i < MAX_HEAP_DUMPS - 1; ++i) {
// Leave the newest `MAX_HEAP_DUMPS - 1` tombstones in place.
existingTombstones.pollLast();
}
for (File file : existingTombstones) {
if (!file.delete()) {
Slog.w("System", "Failed to clean up hprof " + file);
}
}
}
try {
String date = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss").format(new Date());
String filename = "/data/system/heapdump/fdtrack-" + date + ".hprof";
Debug.dumpHprofData(filename);
} catch (IOException ex) {
Slog.e("System", "Failed to dump fdtrack hprof", ex);
}
} |
Dump system_server's heap.
For privacy reasons, these aren't automatically pulled into bugreports:
they must be manually pulled by the user.
| SystemServer::dumpHprof | java | Reginer/aosp-android-jar | android-33/src/com/android/server/SystemServer.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/server/SystemServer.java | MIT |
private static void spawnFdLeakCheckThread() {
final int enableThreshold = SystemProperties.getInt(SYSPROP_FDTRACK_ENABLE_THRESHOLD, 1024);
final int abortThreshold = SystemProperties.getInt(SYSPROP_FDTRACK_ABORT_THRESHOLD, 2048);
final int checkInterval = SystemProperties.getInt(SYSPROP_FDTRACK_INTERVAL, 120);
new Thread(() -> {
boolean enabled = false;
long nextWrite = 0;
while (true) {
int maxFd = getMaxFd();
if (maxFd > enableThreshold) {
// Do a manual GC to clean up fds that are hanging around as garbage.
System.gc();
System.runFinalization();
maxFd = getMaxFd();
}
if (maxFd > enableThreshold && !enabled) {
Slog.i("System", "fdtrack enable threshold reached, enabling");
FrameworkStatsLog.write(FrameworkStatsLog.FDTRACK_EVENT_OCCURRED,
FrameworkStatsLog.FDTRACK_EVENT_OCCURRED__EVENT__ENABLED,
maxFd);
System.loadLibrary("fdtrack");
enabled = true;
} else if (maxFd > abortThreshold) {
Slog.i("System", "fdtrack abort threshold reached, dumping and aborting");
FrameworkStatsLog.write(FrameworkStatsLog.FDTRACK_EVENT_OCCURRED,
FrameworkStatsLog.FDTRACK_EVENT_OCCURRED__EVENT__ABORTING,
maxFd);
dumpHprof();
fdtrackAbort();
} else {
// Limit this to once per hour.
long now = SystemClock.elapsedRealtime();
if (now > nextWrite) {
nextWrite = now + 60 * 60 * 1000;
FrameworkStatsLog.write(FrameworkStatsLog.FDTRACK_EVENT_OCCURRED,
enabled ? FrameworkStatsLog.FDTRACK_EVENT_OCCURRED__EVENT__ENABLED
: FrameworkStatsLog.FDTRACK_EVENT_OCCURRED__EVENT__DISABLED,
maxFd);
}
}
try {
Thread.sleep(checkInterval * 1000);
} catch (InterruptedException ex) {
continue;
}
}
}).start();
} |
Spawn a thread that monitors for fd leaks.
| SystemServer::spawnFdLeakCheckThread | java | Reginer/aosp-android-jar | android-33/src/com/android/server/SystemServer.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/server/SystemServer.java | MIT |
public static void main(String[] args) {
new SystemServer().run();
} |
The main entry point from zygote.
| SystemServer::main | java | Reginer/aosp-android-jar | android-33/src/com/android/server/SystemServer.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/server/SystemServer.java | MIT |
public BarViewInfo(Drawable icon, @IntRange(from = 0) int barHeight,
@Nullable CharSequence title, CharSequence summary,
@Nullable CharSequence contentDescription) {
mIcon = icon;
mHeight = barHeight;
mTitle = title;
mSummary = summary;
mContentDescription = contentDescription;
} |
Construct a BarViewInfo instance.
@param icon The icon of bar view.
@param barHeight The height of bar view. Larger number shows a higher bar view.
@param title The string for title. If this is null, use the height of the bar.
@param summary The string for summary.
@param contentDescription Optional text that briefly describes the contents of the icon.
| BarViewInfo::BarViewInfo | java | Reginer/aosp-android-jar | android-33/src/com/android/settingslib/widget/BarViewInfo.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/settingslib/widget/BarViewInfo.java | MIT |
public void setClickListener(@Nullable View.OnClickListener listener) {
mClickListener = listener;
} |
Set a click listener for bar view.
| BarViewInfo::setClickListener | java | Reginer/aosp-android-jar | android-33/src/com/android/settingslib/widget/BarViewInfo.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/settingslib/widget/BarViewInfo.java | MIT |
public synchronized void setDnsServersOn(LinkProperties lp) {
lp.setDnsServers(mCurrentServers);
} |
Indexes the servers so we can update their lifetimes more quickly in the common case where
servers are not being added, but only being refreshed.
private HashMap<InetAddress, DnsServerEntry> mIndex;
public DnsServerRepository() {
mCurrentServers = new HashSet();
mAllServers = new ArrayList<DnsServerEntry>(NUM_SERVERS);
mIndex = new HashMap<InetAddress, DnsServerEntry>(NUM_SERVERS);
}
/** Sets the DNS servers of the provided LinkProperties object to the current servers. | DnsServerRepository::setDnsServersOn | java | Reginer/aosp-android-jar | android-32/src/com/android/server/net/NetlinkTracker.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/net/NetlinkTracker.java | MIT |
public synchronized boolean addServers(long lifetime, String[] addresses) {
// The lifetime is actually an unsigned 32-bit number, but Java doesn't have unsigned.
// Technically 0xffffffff (the maximum) is special and means "forever", but 2^32 seconds
// (136 years) is close enough.
long now = System.currentTimeMillis();
long expiry = now + 1000 * lifetime;
// Go through the list of servers. For each one, update the entry if one exists, and
// create one if it doesn't.
for (String addressString : addresses) {
InetAddress address;
try {
address = InetAddress.parseNumericAddress(addressString);
} catch (IllegalArgumentException ex) {
continue;
}
if (!updateExistingEntry(address, expiry)) {
// There was no entry for this server. Create one, unless it's already expired
// (i.e., if the lifetime is zero; it cannot be < 0 because it's unsigned).
if (expiry > now) {
DnsServerEntry entry = new DnsServerEntry(address, expiry);
mAllServers.add(entry);
mIndex.put(address, entry);
}
}
}
// Sort the servers by expiry.
Collections.sort(mAllServers);
// Prune excess entries and update the current server list.
return updateCurrentServers();
} |
Notifies the class of new DNS server information.
@param lifetime the time in seconds that the DNS servers are valid.
@param addresses the string representations of the IP addresses of the DNS servers to use.
| DnsServerRepository::addServers | java | Reginer/aosp-android-jar | android-32/src/com/android/server/net/NetlinkTracker.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/net/NetlinkTracker.java | MIT |
public EthernetNetworkManagementException(@NonNull final String errorMessage) {
super(errorMessage);
} | /*
Copyright (C) 2021 The Android Open Source Project
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
package android.net;
import android.annotation.NonNull;
import android.annotation.SystemApi;
import android.os.Parcel;
import android.os.Parcelable;
import java.util.Objects;
/** @hide
@SystemApi
public final class EthernetNetworkManagementException
extends RuntimeException implements Parcelable {
/* @hide | EthernetNetworkManagementException::EthernetNetworkManagementException | java | Reginer/aosp-android-jar | android-35/src/android/net/EthernetNetworkManagementException.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/android/net/EthernetNetworkManagementException.java | MIT |
public Builder(@NonNull CharSequence title) {
mTitle = requireNonNull(title);
} |
Creates a {@link Builder} for a {@link SafetyCenterEntry}.
@param title a title that describes this static entry
| Builder::Builder | java | Reginer/aosp-android-jar | android-35/src/android/safetycenter/SafetyCenterStaticEntry.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/android/safetycenter/SafetyCenterStaticEntry.java | MIT |
public Builder(@NonNull SafetyCenterStaticEntry safetyCenterStaticEntry) {
mTitle = safetyCenterStaticEntry.mTitle;
mSummary = safetyCenterStaticEntry.mSummary;
mPendingIntent = safetyCenterStaticEntry.mPendingIntent;
} |
Creates a {@link Builder} with the values from the given {@link SafetyCenterStaticEntry}.
| Builder::Builder | java | Reginer/aosp-android-jar | android-35/src/android/safetycenter/SafetyCenterStaticEntry.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/android/safetycenter/SafetyCenterStaticEntry.java | MIT |
default void onUnlocked() {} |
Scan locked the signal.
It can also be notified after signal is locked if the signal attributes transmission
parameter of the signal is changed (e.g., Modulation).
void onLocked();
/** Scan unlocked the signal. | onUnlocked | java | Reginer/aosp-android-jar | android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | MIT |
default void onFrequenciesLongReported(@NonNull long[] frequencies) {
final int[] intFrequencies = new int[frequencies.length];
for (int i = 0; i < frequencies.length; i++) {
intFrequencies[i] = (int) frequencies[i];
}
onFrequenciesReported(intFrequencies);
} |
@deprecated Use {@link #onFrequenciesLongReported(long[])}
@Deprecated void onFrequenciesReported(@NonNull int[] frequencies);
/** Signal frequencies in Hertz | onFrequenciesLongReported | java | Reginer/aosp-android-jar | android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | MIT |
default void onModulationReported(@FrontendStatus.FrontendModulation int modulation) {} |
@deprecated Use {@link #onFrequenciesLongReported(long[])}
@Deprecated void onFrequenciesReported(@NonNull int[] frequencies);
/** Signal frequencies in Hertz
default void onFrequenciesLongReported(@NonNull long[] frequencies) {
final int[] intFrequencies = new int[frequencies.length];
for (int i = 0; i < frequencies.length; i++) {
intFrequencies[i] = (int) frequencies[i];
}
onFrequenciesReported(intFrequencies);
}
/** Symbols per second
void onSymbolRatesReported(@NonNull int[] rate);
/** Locked Plp Ids for DVBT2 frontend.
void onPlpIdsReported(@NonNull int[] plpIds);
/** Locked group Ids for DVBT2 frontend.
void onGroupIdsReported(@NonNull int[] groupIds);
/** Stream Ids.
void onInputStreamIdsReported(@NonNull int[] inputStreamIds);
/** Locked signal standard for DVBS.
void onDvbsStandardReported(@DvbsFrontendSettings.Standard int dvbsStandard);
/** Locked signal standard. for DVBT
void onDvbtStandardReported(@DvbtFrontendSettings.Standard int dvbtStandard);
/** Locked signal SIF standard for Analog.
void onAnalogSifStandardReported(@AnalogFrontendSettings.SifStandard int sif);
/** PLP status in a tuned frequency band for ATSC3 frontend.
void onAtsc3PlpInfosReported(@NonNull Atsc3PlpInfo[] atsc3PlpInfos);
/** Frontend hierarchy.
void onHierarchyReported(@DvbtFrontendSettings.Hierarchy int hierarchy);
/** Frontend signal type.
void onSignalTypeReported(@AnalogFrontendSettings.SignalType int signalType);
/** Frontend modulation reported. | onModulationReported | java | Reginer/aosp-android-jar | android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | MIT |
default void onPriorityReported(boolean isHighPriority) {} |
@deprecated Use {@link #onFrequenciesLongReported(long[])}
@Deprecated void onFrequenciesReported(@NonNull int[] frequencies);
/** Signal frequencies in Hertz
default void onFrequenciesLongReported(@NonNull long[] frequencies) {
final int[] intFrequencies = new int[frequencies.length];
for (int i = 0; i < frequencies.length; i++) {
intFrequencies[i] = (int) frequencies[i];
}
onFrequenciesReported(intFrequencies);
}
/** Symbols per second
void onSymbolRatesReported(@NonNull int[] rate);
/** Locked Plp Ids for DVBT2 frontend.
void onPlpIdsReported(@NonNull int[] plpIds);
/** Locked group Ids for DVBT2 frontend.
void onGroupIdsReported(@NonNull int[] groupIds);
/** Stream Ids.
void onInputStreamIdsReported(@NonNull int[] inputStreamIds);
/** Locked signal standard for DVBS.
void onDvbsStandardReported(@DvbsFrontendSettings.Standard int dvbsStandard);
/** Locked signal standard. for DVBT
void onDvbtStandardReported(@DvbtFrontendSettings.Standard int dvbtStandard);
/** Locked signal SIF standard for Analog.
void onAnalogSifStandardReported(@AnalogFrontendSettings.SifStandard int sif);
/** PLP status in a tuned frequency band for ATSC3 frontend.
void onAtsc3PlpInfosReported(@NonNull Atsc3PlpInfo[] atsc3PlpInfos);
/** Frontend hierarchy.
void onHierarchyReported(@DvbtFrontendSettings.Hierarchy int hierarchy);
/** Frontend signal type.
void onSignalTypeReported(@AnalogFrontendSettings.SignalType int signalType);
/** Frontend modulation reported.
default void onModulationReported(@FrontendStatus.FrontendModulation int modulation) {}
/** Frontend scan message priority reported. | onPriorityReported | java | Reginer/aosp-android-jar | android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | MIT |
default void onDvbcAnnexReported(@DvbcFrontendSettings.Annex int dvbcAnnex) {} |
@deprecated Use {@link #onFrequenciesLongReported(long[])}
@Deprecated void onFrequenciesReported(@NonNull int[] frequencies);
/** Signal frequencies in Hertz
default void onFrequenciesLongReported(@NonNull long[] frequencies) {
final int[] intFrequencies = new int[frequencies.length];
for (int i = 0; i < frequencies.length; i++) {
intFrequencies[i] = (int) frequencies[i];
}
onFrequenciesReported(intFrequencies);
}
/** Symbols per second
void onSymbolRatesReported(@NonNull int[] rate);
/** Locked Plp Ids for DVBT2 frontend.
void onPlpIdsReported(@NonNull int[] plpIds);
/** Locked group Ids for DVBT2 frontend.
void onGroupIdsReported(@NonNull int[] groupIds);
/** Stream Ids.
void onInputStreamIdsReported(@NonNull int[] inputStreamIds);
/** Locked signal standard for DVBS.
void onDvbsStandardReported(@DvbsFrontendSettings.Standard int dvbsStandard);
/** Locked signal standard. for DVBT
void onDvbtStandardReported(@DvbtFrontendSettings.Standard int dvbtStandard);
/** Locked signal SIF standard for Analog.
void onAnalogSifStandardReported(@AnalogFrontendSettings.SifStandard int sif);
/** PLP status in a tuned frequency band for ATSC3 frontend.
void onAtsc3PlpInfosReported(@NonNull Atsc3PlpInfo[] atsc3PlpInfos);
/** Frontend hierarchy.
void onHierarchyReported(@DvbtFrontendSettings.Hierarchy int hierarchy);
/** Frontend signal type.
void onSignalTypeReported(@AnalogFrontendSettings.SignalType int signalType);
/** Frontend modulation reported.
default void onModulationReported(@FrontendStatus.FrontendModulation int modulation) {}
/** Frontend scan message priority reported.
default void onPriorityReported(boolean isHighPriority) {}
/** DVBC Frontend Annex reported. | onDvbcAnnexReported | java | Reginer/aosp-android-jar | android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | MIT |
default void onDvbtCellIdsReported(@NonNull int[] dvbtCellIds) {} |
@deprecated Use {@link #onFrequenciesLongReported(long[])}
@Deprecated void onFrequenciesReported(@NonNull int[] frequencies);
/** Signal frequencies in Hertz
default void onFrequenciesLongReported(@NonNull long[] frequencies) {
final int[] intFrequencies = new int[frequencies.length];
for (int i = 0; i < frequencies.length; i++) {
intFrequencies[i] = (int) frequencies[i];
}
onFrequenciesReported(intFrequencies);
}
/** Symbols per second
void onSymbolRatesReported(@NonNull int[] rate);
/** Locked Plp Ids for DVBT2 frontend.
void onPlpIdsReported(@NonNull int[] plpIds);
/** Locked group Ids for DVBT2 frontend.
void onGroupIdsReported(@NonNull int[] groupIds);
/** Stream Ids.
void onInputStreamIdsReported(@NonNull int[] inputStreamIds);
/** Locked signal standard for DVBS.
void onDvbsStandardReported(@DvbsFrontendSettings.Standard int dvbsStandard);
/** Locked signal standard. for DVBT
void onDvbtStandardReported(@DvbtFrontendSettings.Standard int dvbtStandard);
/** Locked signal SIF standard for Analog.
void onAnalogSifStandardReported(@AnalogFrontendSettings.SifStandard int sif);
/** PLP status in a tuned frequency band for ATSC3 frontend.
void onAtsc3PlpInfosReported(@NonNull Atsc3PlpInfo[] atsc3PlpInfos);
/** Frontend hierarchy.
void onHierarchyReported(@DvbtFrontendSettings.Hierarchy int hierarchy);
/** Frontend signal type.
void onSignalTypeReported(@AnalogFrontendSettings.SignalType int signalType);
/** Frontend modulation reported.
default void onModulationReported(@FrontendStatus.FrontendModulation int modulation) {}
/** Frontend scan message priority reported.
default void onPriorityReported(boolean isHighPriority) {}
/** DVBC Frontend Annex reported.
default void onDvbcAnnexReported(@DvbcFrontendSettings.Annex int dvbcAnnex) {}
/** DVBT Frontend Cell Ids reported. | onDvbtCellIdsReported | java | Reginer/aosp-android-jar | android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/media/tv/tuner/frontend/ScanCallback.java | MIT |
public ArrayMapWithHistory(@IntRange(from = 1) int maxHistorySize) {
if (maxHistorySize < 1) {
throw new IllegalArgumentException("maxHistorySize < 1: " + maxHistorySize);
}
mMaxHistorySize = maxHistorySize;
} |
Creates an instance that records, at most, the specified number of values against each key.
| ArrayMapWithHistory::ArrayMapWithHistory | java | Reginer/aosp-android-jar | android-32/src/com/android/server/timezonedetector/ArrayMapWithHistory.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/timezonedetector/ArrayMapWithHistory.java | MIT |
public int size() {
return mMap == null ? 0 : mMap.size();
} |
See {@link ArrayMap#size()}.
| ArrayMapWithHistory::size | java | Reginer/aosp-android-jar | android-32/src/com/android/server/timezonedetector/ArrayMapWithHistory.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/timezonedetector/ArrayMapWithHistory.java | MIT |
public void dump(@NonNull IndentingPrintWriter ipw) {
if (mMap == null) {
ipw.println("{Empty}");
} else {
for (int i = 0; i < mMap.size(); i++) {
ipw.println("key idx: " + i + "=" + mMap.keyAt(i));
ReferenceWithHistory<V> value = mMap.valueAt(i);
ipw.println("val idx: " + i + "=" + value);
ipw.increaseIndent();
ipw.println("Historic values=[");
ipw.increaseIndent();
value.dump(ipw);
ipw.decreaseIndent();
ipw.println("]");
ipw.decreaseIndent();
}
}
ipw.flush();
} |
Dumps the content of the map, including historic values, using the supplied writer.
| ArrayMapWithHistory::dump | java | Reginer/aosp-android-jar | android-32/src/com/android/server/timezonedetector/ArrayMapWithHistory.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/timezonedetector/ArrayMapWithHistory.java | MIT |
public AESWrapEngine()
{
super(new AESEngine());
} |
Create a regular AESWrapEngine specifying the encrypt for wrapping, decrypt for unwrapping.
| AESWrapEngine::AESWrapEngine | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/org/bouncycastle/crypto/engines/AESWrapEngine.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/org/bouncycastle/crypto/engines/AESWrapEngine.java | MIT |
public AESWrapEngine(boolean useReverseDirection)
{
super(new AESEngine(), useReverseDirection);
} |
Create an AESWrapEngine where the underlying cipher is set to decrypt for wrapping, encrypt for unwrapping.
@param useReverseDirection true if underlying cipher should be used in decryption mode, false otherwise.
| AESWrapEngine::AESWrapEngine | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/org/bouncycastle/crypto/engines/AESWrapEngine.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/org/bouncycastle/crypto/engines/AESWrapEngine.java | MIT |
public FastPrintWriter(OutputStream out, boolean autoFlush) {
this(out, autoFlush, 8192);
} |
Constructs a new {@code PrintWriter} with {@code out} as its target
stream. The parameter {@code autoFlush} determines if the print writer
automatically flushes its contents to the target stream when a newline is
encountered.
@param out
the target output stream.
@param autoFlush
indicates whether contents are flushed upon encountering a
newline sequence.
@throws NullPointerException
if {@code out} is {@code null}.
| DummyWriter::FastPrintWriter | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public FastPrintWriter(OutputStream out, boolean autoFlush, int bufferLen) {
super(new DummyWriter(), autoFlush);
if (out == null) {
throw new NullPointerException("out is null");
}
mBufferLen = bufferLen;
mText = new char[bufferLen];
mBytes = ByteBuffer.allocate(mBufferLen);
mOutputStream = out;
mWriter = null;
mPrinter = null;
mAutoFlush = autoFlush;
mSeparator = System.lineSeparator();
initDefaultEncoder();
} |
Constructs a new {@code PrintWriter} with {@code out} as its target
stream and a custom buffer size. The parameter {@code autoFlush} determines
if the print writer automatically flushes its contents to the target stream
when a newline is encountered.
@param out
the target output stream.
@param autoFlush
indicates whether contents are flushed upon encountering a
newline sequence.
@param bufferLen
specifies the size of the FastPrintWriter's internal buffer; the
default is 8192.
@throws NullPointerException
if {@code out} is {@code null}.
| DummyWriter::FastPrintWriter | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public FastPrintWriter(Writer wr) {
this(wr, false, 8192);
} |
Constructs a new {@code PrintWriter} with {@code wr} as its target
writer. By default, the new print writer does not automatically flush its
contents to the target writer when a newline is encountered.
<p>NOTE: Unlike PrintWriter, this version will still do buffering inside of
FastPrintWriter before sending data to the Writer. This means you must call
flush() before retrieving any data from the Writer.</p>
@param wr
the target writer.
@throws NullPointerException
if {@code wr} is {@code null}.
| DummyWriter::FastPrintWriter | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public FastPrintWriter(Writer wr, boolean autoFlush) {
this(wr, autoFlush, 8192);
} |
Constructs a new {@code PrintWriter} with {@code wr} as its target
writer. The parameter {@code autoFlush} determines if the print writer
automatically flushes its contents to the target writer when a newline is
encountered.
@param wr
the target writer.
@param autoFlush
indicates whether to flush contents upon encountering a
newline sequence.
@throws NullPointerException
if {@code out} is {@code null}.
| DummyWriter::FastPrintWriter | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public FastPrintWriter(Writer wr, boolean autoFlush, int bufferLen) {
super(new DummyWriter(), autoFlush);
if (wr == null) {
throw new NullPointerException("wr is null");
}
mBufferLen = bufferLen;
mText = new char[bufferLen];
mBytes = null;
mOutputStream = null;
mWriter = wr;
mPrinter = null;
mAutoFlush = autoFlush;
mSeparator = System.lineSeparator();
initDefaultEncoder();
} |
Constructs a new {@code PrintWriter} with {@code wr} as its target
writer and a custom buffer size. The parameter {@code autoFlush} determines
if the print writer automatically flushes its contents to the target writer
when a newline is encountered.
@param wr
the target writer.
@param autoFlush
indicates whether to flush contents upon encountering a
newline sequence.
@param bufferLen
specifies the size of the FastPrintWriter's internal buffer; the
default is 8192.
@throws NullPointerException
if {@code wr} is {@code null}.
| DummyWriter::FastPrintWriter | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public FastPrintWriter(Printer pr) {
this(pr, 512);
} |
Constructs a new {@code PrintWriter} with {@code pr} as its target
printer and the default buffer size. Because a {@link Printer} is line-base,
autoflush is always enabled.
@param pr
the target writer.
@throws NullPointerException
if {@code pr} is {@code null}.
| DummyWriter::FastPrintWriter | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public FastPrintWriter(Printer pr, int bufferLen) {
super(new DummyWriter(), true);
if (pr == null) {
throw new NullPointerException("pr is null");
}
mBufferLen = bufferLen;
mText = new char[bufferLen];
mBytes = null;
mOutputStream = null;
mWriter = null;
mPrinter = pr;
mAutoFlush = true;
mSeparator = System.lineSeparator();
initDefaultEncoder();
} |
Constructs a new {@code PrintWriter} with {@code pr} as its target
printer and a custom buffer size. Because a {@link Printer} is line-base,
autoflush is always enabled.
@param pr
the target writer.
@param bufferLen
specifies the size of the FastPrintWriter's internal buffer; the
default is 512.
@throws NullPointerException
if {@code pr} is {@code null}.
| DummyWriter::FastPrintWriter | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public boolean checkError() {
flush();
synchronized (lock) {
return mIoError;
}
} |
Flushes this writer and returns the value of the error flag.
@return {@code true} if either an {@code IOException} has been thrown
previously or if {@code setError()} has been called;
{@code false} otherwise.
@see #setError()
| DummyWriter::checkError | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
protected void clearError() {
synchronized (lock) {
mIoError = false;
}
} |
Sets the error state of the stream to false.
@since 1.6
| DummyWriter::clearError | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
protected void setError() {
synchronized (lock) {
mIoError = true;
}
} |
Sets the error flag of this writer to true.
| DummyWriter::setError | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public void print(char[] charArray) {
synchronized (lock) {
try {
appendLocked(charArray, 0, charArray.length);
} catch (IOException e) {
Log.w("FastPrintWriter", "Write failure", e);
setError();
}
}
} |
Prints the string representation of the specified character array
to the target.
@param charArray
the character array to print to the target.
@see #print(String)
| DummyWriter::print | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public void print(char ch) {
synchronized (lock) {
try {
appendLocked(ch);
} catch (IOException e) {
Log.w("FastPrintWriter", "Write failure", e);
setError();
}
}
} |
Prints the string representation of the specified character to the
target.
@param ch
the character to print to the target.
@see #print(String)
| DummyWriter::print | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public void print(String str) {
if (str == null) {
str = String.valueOf((Object) null);
}
synchronized (lock) {
try {
appendLocked(str, 0, str.length());
} catch (IOException e) {
Log.w("FastPrintWriter", "Write failure", e);
setError();
}
}
} |
Prints a string to the target. The string is converted to an array of
bytes using the encoding chosen during the construction of this writer.
The bytes are then written to the target with {@code write(int)}.
<p>
If an I/O error occurs, this writer's error flag is set to {@code true}.
@param str
the string to print to the target.
@see #write(int)
| DummyWriter::print | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public void println() {
synchronized (lock) {
try {
appendLocked(mSeparator, 0, mSeparator.length());
if (mAutoFlush) {
flushLocked();
}
} catch (IOException e) {
Log.w("FastPrintWriter", "Write failure", e);
setError();
}
}
} |
Prints a newline. Flushes this writer if the autoFlush flag is set to {@code true}.
| DummyWriter::println | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public void println(char[] chars) {
print(chars);
println();
} |
Prints the string representation of the character array {@code chars} followed by a newline.
Flushes this writer if the autoFlush flag is set to {@code true}.
| DummyWriter::println | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public void println(char c) {
print(c);
println();
} |
Prints the string representation of the char {@code c} followed by a newline.
Flushes this writer if the autoFlush flag is set to {@code true}.
| DummyWriter::println | java | Reginer/aosp-android-jar | android-35/src/com/android/internal/util/FastPrintWriter.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/internal/util/FastPrintWriter.java | MIT |
public void acknowledgeRequest(int serial) {
mRil.processRequestAck(serial);
} |
Acknowledge the receipt of radio request sent to the vendor. This must be sent only for
radio request which take long time to respond.
For more details, refer https://source.android.com/devices/tech/connect/ril.html
@param serial Serial no. of the request whose acknowledgement is sent.
| DataResponse::acknowledgeRequest | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void allocatePduSessionIdResponse(RadioResponseInfo responseInfo, int id) {
RILRequest rr = mRil.processResponse(HAL_SERVICE_DATA, responseInfo);
if (rr != null) {
if (responseInfo.error == RadioError.NONE) {
RadioResponse.sendMessageResponse(rr.mResult, id);
}
mRil.processResponseDone(rr, responseInfo, id);
}
} |
@param responseInfo Response info struct containing response type, serial no. and error
@param id The pdu session id allocated
| DataResponse::allocatePduSessionIdResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void cancelHandoverResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::cancelHandoverResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void deactivateDataCallResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::deactivateDataCallResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void getDataCallListResponse(RadioResponseInfo responseInfo,
android.hardware.radio.data.SetupDataCallResult[] dataCallResultList) {
RILRequest rr = mRil.processResponse(HAL_SERVICE_DATA, responseInfo);
if (rr != null) {
ArrayList<DataCallResponse> response =
RILUtils.convertHalDataCallResultList(dataCallResultList);
if (responseInfo.error == RadioError.NONE) {
RadioResponse.sendMessageResponse(rr.mResult, response);
}
mRil.processResponseDone(rr, responseInfo, response);
}
} |
@param responseInfo Response info struct containing response type, serial no. and error
@param dataCallResultList Response to get data call list as defined by SetupDataCallResult
| DataResponse::getDataCallListResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void getSlicingConfigResponse(RadioResponseInfo responseInfo,
android.hardware.radio.data.SlicingConfig slicingConfig) {
RILRequest rr = mRil.processResponse(HAL_SERVICE_DATA, responseInfo);
if (rr != null) {
NetworkSlicingConfig ret = RILUtils.convertHalSlicingConfig(slicingConfig);
if (responseInfo.error == RadioError.NONE) {
RadioResponse.sendMessageResponse(rr.mResult, ret);
}
mRil.processResponseDone(rr, responseInfo, ret);
}
} |
@param responseInfo Response info struct containing response type, serial no. and error
@param slicingConfig Current slicing configuration
| DataResponse::getSlicingConfigResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void releasePduSessionIdResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::releasePduSessionIdResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void setDataAllowedResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::setDataAllowedResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void setDataProfileResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::setDataProfileResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void setDataThrottlingResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::setDataThrottlingResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void setInitialAttachApnResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::setInitialAttachApnResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void setupDataCallResponse(RadioResponseInfo responseInfo,
android.hardware.radio.data.SetupDataCallResult setupDataCallResult) {
RILRequest rr = mRil.processResponse(HAL_SERVICE_DATA, responseInfo);
if (rr != null) {
DataCallResponse response = RILUtils.convertHalDataCallResult(setupDataCallResult);
if (responseInfo.error == RadioError.NONE) {
RadioResponse.sendMessageResponse(rr.mResult, response);
}
mRil.processResponseDone(rr, responseInfo, response);
}
} |
@param responseInfo Response info struct containing response type, serial no. and error
@param setupDataCallResult Response to data call setup as defined by SetupDataCallResult
| DataResponse::setupDataCallResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void startHandoverResponse(RadioResponseInfo responseInfo) {
RadioResponse.responseVoid(HAL_SERVICE_DATA, mRil, responseInfo);
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::startHandoverResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void startKeepaliveResponse(RadioResponseInfo responseInfo,
android.hardware.radio.data.KeepaliveStatus keepaliveStatus) {
RILRequest rr = mRil.processResponse(HAL_SERVICE_DATA, responseInfo);
if (rr == null) return;
KeepaliveStatus ret = null;
try {
switch(responseInfo.error) {
case RadioError.NONE:
int convertedStatus = RILUtils.convertHalKeepaliveStatusCode(
keepaliveStatus.code);
if (convertedStatus < 0) {
ret = new KeepaliveStatus(KeepaliveStatus.ERROR_UNSUPPORTED);
} else {
ret = new KeepaliveStatus(
keepaliveStatus.sessionHandle, convertedStatus);
}
// If responseInfo.error is NONE, response function sends the response message
// even if result is actually an error.
RadioResponse.sendMessageResponse(rr.mResult, ret);
break;
case RadioError.REQUEST_NOT_SUPPORTED:
ret = new KeepaliveStatus(KeepaliveStatus.ERROR_UNSUPPORTED);
break;
case RadioError.NO_RESOURCES:
ret = new KeepaliveStatus(KeepaliveStatus.ERROR_NO_RESOURCES);
break;
default:
ret = new KeepaliveStatus(KeepaliveStatus.ERROR_UNKNOWN);
break;
}
} finally {
// If responseInfo.error != NONE, the processResponseDone sends the response message.
mRil.processResponseDone(rr, responseInfo, ret);
}
} |
@param responseInfo Response info struct containing response type, serial no. and error
@param keepaliveStatus status of the keepalive with a handle for the session
| DataResponse::startKeepaliveResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public void stopKeepaliveResponse(RadioResponseInfo responseInfo) {
RILRequest rr = mRil.processResponse(HAL_SERVICE_DATA, responseInfo);
if (rr == null) return;
try {
if (responseInfo.error == RadioError.NONE) {
RadioResponse.sendMessageResponse(rr.mResult, null);
} else {
//TODO: Error code translation
}
} finally {
mRil.processResponseDone(rr, responseInfo, null);
}
} |
@param responseInfo Response info struct containing response type, serial no. and error
| DataResponse::stopKeepaliveResponse | java | Reginer/aosp-android-jar | android-34/src/com/android/internal/telephony/DataResponse.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/internal/telephony/DataResponse.java | MIT |
public DrmInfoEvent(int uniqueId, int type, String message) {
super(uniqueId, type, message);
checkTypeValidity(type);
} |
Creates a <code>DrmInfoEvent</code> object with the specified parameters.
@param uniqueId Unique session identifier.
@param type Type of the event. Must be any of the event types defined above,
or the constants defined in {@link DrmEvent}.
@param message Message description. It can be null.
| DrmInfoEvent::DrmInfoEvent | java | Reginer/aosp-android-jar | android-34/src/android/drm/DrmInfoEvent.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/drm/DrmInfoEvent.java | MIT |
public DrmInfoEvent(int uniqueId, int type, String message,
HashMap<String, Object> attributes) {
super(uniqueId, type, message, attributes);
checkTypeValidity(type);
} |
Creates a <code>DrmInfoEvent</code> object with the specified parameters.
@param uniqueId Unique session identifier.
@param type Type of the event. Must be any of the event types defined above,
or the constants defined in {@link DrmEvent}
@param message Message description. It can be null.
@param attributes Attributes for extensible information. Could be any
information provided by the plug-in.
| DrmInfoEvent::DrmInfoEvent | java | Reginer/aosp-android-jar | android-34/src/android/drm/DrmInfoEvent.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/drm/DrmInfoEvent.java | MIT |
private void checkTypeValidity(int type) {
if (type < TYPE_ALREADY_REGISTERED_BY_ANOTHER_ACCOUNT ||
type > TYPE_RIGHTS_REMOVED) {
if (type != TYPE_ALL_RIGHTS_REMOVED &&
type != TYPE_DRM_INFO_PROCESSED) {
final String msg = "Unsupported type: " + type;
throw new IllegalArgumentException(msg);
}
}
} |
Creates a <code>DrmInfoEvent</code> object with the specified parameters.
@param uniqueId Unique session identifier.
@param type Type of the event. Must be any of the event types defined above,
or the constants defined in {@link DrmEvent}
@param message Message description. It can be null.
@param attributes Attributes for extensible information. Could be any
information provided by the plug-in.
public DrmInfoEvent(int uniqueId, int type, String message,
HashMap<String, Object> attributes) {
super(uniqueId, type, message, attributes);
checkTypeValidity(type);
}
/*
Check the validity of the given type.
To overcome a design flaw, we need also accept the type constants
defined in super class, DrmEvent.
| DrmInfoEvent::checkTypeValidity | java | Reginer/aosp-android-jar | android-34/src/android/drm/DrmInfoEvent.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/drm/DrmInfoEvent.java | MIT |
public boolean wouldLaunchResolverActivity(Intent intent, int currentUserId) {
ActivityInfo targetActivityInfo = getTargetActivityInfo(intent, currentUserId,
false /* onlyDirectBootAware */);
return targetActivityInfo == null;
} |
Determines if sending the given intent would result in starting an Intent resolver activity,
instead of resolving to a specific component.
@param intent the intent
@param currentUserId the id for the user to resolve as
@return true if the intent would launch a resolver activity
| ActivityIntentHelper::wouldLaunchResolverActivity | java | Reginer/aosp-android-jar | android-33/src/com/android/systemui/ActivityIntentHelper.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/systemui/ActivityIntentHelper.java | MIT |
public ActivityInfo getTargetActivityInfo(Intent intent, int currentUserId,
boolean onlyDirectBootAware) {
PackageManager packageManager = mContext.getPackageManager();
int flags = PackageManager.MATCH_DEFAULT_ONLY;
if (!onlyDirectBootAware) {
flags |= PackageManager.MATCH_DIRECT_BOOT_AWARE
| PackageManager.MATCH_DIRECT_BOOT_UNAWARE;
}
final List<ResolveInfo> appList = packageManager.queryIntentActivitiesAsUser(
intent, flags, currentUserId);
if (appList.size() == 0) {
return null;
}
ResolveInfo resolved = packageManager.resolveActivityAsUser(intent,
flags | PackageManager.GET_META_DATA, currentUserId);
if (resolved == null || wouldLaunchResolverActivity(resolved, appList)) {
return null;
} else {
return resolved.activityInfo;
}
} |
Returns info about the target Activity of a given intent, or null if the intent does not
resolve to a specific component meeting the requirements.
@param onlyDirectBootAware a boolean indicating whether the matched activity packages must
be direct boot aware when in direct boot mode if false, all packages are considered
a match even if they are not aware.
@return the target activity info of the intent it resolves to a specific package or
{@code null} if it resolved to the resolver activity
| ActivityIntentHelper::getTargetActivityInfo | java | Reginer/aosp-android-jar | android-33/src/com/android/systemui/ActivityIntentHelper.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/systemui/ActivityIntentHelper.java | MIT |
public boolean wouldShowOverLockscreen(Intent intent, int currentUserId) {
ActivityInfo targetActivityInfo = getTargetActivityInfo(intent,
currentUserId, false /* onlyDirectBootAware */);
return targetActivityInfo != null
&& (targetActivityInfo.flags & (ActivityInfo.FLAG_SHOW_WHEN_LOCKED
| ActivityInfo.FLAG_SHOW_FOR_ALL_USERS)) > 0;
} |
Determines if the given intent resolves to an Activity which is allowed to appear above
the lock screen.
@param intent the intent to resolve
@return true if the launched Activity would appear above the lock screen
| ActivityIntentHelper::wouldShowOverLockscreen | java | Reginer/aosp-android-jar | android-33/src/com/android/systemui/ActivityIntentHelper.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/systemui/ActivityIntentHelper.java | MIT |
public boolean wouldLaunchResolverActivity(ResolveInfo resolved, List<ResolveInfo> appList) {
// If the list contains the above resolved activity, then it can't be
// ResolverActivity itself.
for (int i = 0; i < appList.size(); i++) {
ResolveInfo tmp = appList.get(i);
if (tmp.activityInfo.name.equals(resolved.activityInfo.name)
&& tmp.activityInfo.packageName.equals(resolved.activityInfo.packageName)) {
return false;
}
}
return true;
} |
Determines if sending the given intent would result in starting an Intent resolver activity,
instead of resolving to a specific component.
@param resolved the resolveInfo for the intent as returned by resolveActivityAsUser
@param appList a list of resolveInfo as returned by queryIntentActivitiesAsUser
@return true if the intent would launch a resolver activity
| ActivityIntentHelper::wouldLaunchResolverActivity | java | Reginer/aosp-android-jar | android-33/src/com/android/systemui/ActivityIntentHelper.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/systemui/ActivityIntentHelper.java | MIT |
protected PipelinedMsgParser() {
super();
} |
default constructor.
| PipelinedMsgParser::PipelinedMsgParser | java | Reginer/aosp-android-jar | android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | MIT |
public PipelinedMsgParser(SIPMessageListener sipMessageListener,
Pipeline in, boolean debug, int maxMessageSize) {
this();
this.sipMessageListener = sipMessageListener;
rawInputStream = in;
this.maxMessageSize = maxMessageSize;
mythread = new Thread(this);
mythread.setName("PipelineThread-" + getNewUid());
} |
Constructor when we are given a message listener and an input stream
(could be a TCP connection or a file)
@param sipMessageListener
Message listener which has methods that get called back from
the parser when a parse is complete
@param in
Input stream from which to read the input.
@param debug
Enable/disable tracing or lexical analyser switch.
| PipelinedMsgParser::PipelinedMsgParser | java | Reginer/aosp-android-jar | android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | MIT |
public void processInput() {
mythread.start();
} |
Start reading and processing input.
| PipelinedMsgParser::processInput | java | Reginer/aosp-android-jar | android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | MIT |
protected Object clone() {
PipelinedMsgParser p = new PipelinedMsgParser();
p.rawInputStream = this.rawInputStream;
p.sipMessageListener = this.sipMessageListener;
Thread mythread = new Thread(p);
mythread.setName("PipelineThread");
return p;
} |
Create a new pipelined parser from an existing one.
@return A new pipelined parser that reads from the same input stream.
| PipelinedMsgParser::clone | java | Reginer/aosp-android-jar | android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | MIT |
private String readLine(InputStream inputStream) throws IOException {
StringBuffer retval = new StringBuffer("");
while (true) {
char ch;
int i = inputStream.read();
if (i == -1) {
throw new IOException("End of stream");
} else
ch = (char) i;
// reduce the available read size by 1 ("size" of a char).
if (this.maxMessageSize > 0) {
this.sizeCounter--;
if (this.sizeCounter <= 0)
throw new IOException("Max size exceeded!");
}
if (ch != '\r')
retval.append(ch);
if (ch == '\n') {
break;
}
}
return retval.toString();
} |
read a line of input (I cannot use buffered reader because we may need to
switch encodings mid-stream!
| PipelinedMsgParser::readLine | java | Reginer/aosp-android-jar | android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | MIT |
public void run() {
Pipeline inputStream = this.rawInputStream;
// inputStream = new MyFilterInputStream(this.rawInputStream);
// I cannot use buffered reader here because we may need to switch
// encodings to read the message body.
try {
while (true) {
this.sizeCounter = this.maxMessageSize;
// this.messageSize = 0;
StringBuffer inputBuffer = new StringBuffer();
if (Debug.parserDebug)
Debug.println("Starting parse!");
String line1;
String line2 = null;
while (true) {
try {
line1 = readLine(inputStream);
// ignore blank lines.
if (line1.equals("\n")) {
if (Debug.parserDebug) {
Debug.println("Discarding blank line. ");
}
continue;
} else
break;
} catch (IOException ex) {
Debug.printStackTrace(ex);
this.rawInputStream.stopTimer();
return;
}
}
inputBuffer.append(line1);
// Guard against bad guys.
this.rawInputStream.startTimer();
Debug.println("Reading Input Stream");
while (true) {
try {
line2 = readLine(inputStream);
inputBuffer.append(line2);
if (line2.trim().equals(""))
break;
} catch (IOException ex) {
this.rawInputStream.stopTimer();
Debug.printStackTrace(ex);
return;
}
}
// Stop the timer that will kill the read.
this.rawInputStream.stopTimer();
inputBuffer.append(line2);
StringMsgParser smp = new StringMsgParser(sipMessageListener);
smp.readBody = false;
SIPMessage sipMessage = null;
try {
if (Debug.debug) {
Debug.println("About to parse : " + inputBuffer.toString());
}
sipMessage = smp.parseSIPMessage(inputBuffer.toString());
if (sipMessage == null) {
this.rawInputStream.stopTimer();
continue;
}
} catch (ParseException ex) {
// Just ignore the parse exception.
Debug.logError("Detected a parse error", ex);
continue;
}
if (Debug.debug) {
Debug.println("Completed parsing message");
}
ContentLength cl = (ContentLength) sipMessage
.getContentLength();
int contentLength = 0;
if (cl != null) {
contentLength = cl.getContentLength();
} else {
contentLength = 0;
}
if (Debug.debug) {
Debug.println("contentLength " + contentLength);
}
if (contentLength == 0) {
sipMessage.removeContent();
} else if (maxMessageSize == 0
|| contentLength < this.sizeCounter) {
byte[] message_body = new byte[contentLength];
int nread = 0;
while (nread < contentLength) {
// Start my starvation timer.
// This ensures that the other end
// writes at least some data in
// or we will close the pipe from
// him. This prevents DOS attack
// that takes up all our connections.
this.rawInputStream.startTimer();
try {
int readlength = inputStream.read(message_body,
nread, contentLength - nread);
if (readlength > 0) {
nread += readlength;
} else {
break;
}
} catch (IOException ex) {
Debug.logError("Exception Reading Content",ex);
break;
} finally {
// Stop my starvation timer.
this.rawInputStream.stopTimer();
}
}
sipMessage.setMessageContent(message_body);
}
// Content length too large - process the message and
// return error from there.
if (sipMessageListener != null) {
try {
sipMessageListener.processMessage(sipMessage);
} catch (Exception ex) {
// fatal error in processing - close the
// connection.
break;
}
}
}
} finally {
try {
inputStream.close();
} catch (IOException e) {
InternalErrorHandler.handleException(e);
}
}
} |
This is input reading thread for the pipelined parser. You feed it input
through the input stream (see the constructor) and it calls back an event
listener interface for message processing or error. It cleans up the
input - dealing with things like line continuation
| PipelinedMsgParser::run | java | Reginer/aosp-android-jar | android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/gov/nist/javax/sip/parser/PipelinedMsgParser.java | MIT |
private static int hexCharToValue(char c) {
if ('0' <= c && c <= '9') {
return c - '0';
}
if ('a' <= c && c <= 'f') {
return 10 + c - 'a';
}
if ('A' <= c && c <= 'F') {
return 10 + c - 'A';
}
return -1;
} |
Interprets a char as hex digits, returning a number from -1 (invalid char) to 15 ('f').
| UriCodec::hexCharToValue | java | Reginer/aosp-android-jar | android-34/src/android/net/UriCodec.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/net/UriCodec.java | MIT |
public static String decode(
String s, boolean convertPlus, Charset charset, boolean throwOnFailure) {
StringBuilder builder = new StringBuilder(s.length());
appendDecoded(builder, s, convertPlus, charset, throwOnFailure);
return builder.toString();
} |
Decode a string according to the rules of this decoder.
- if {@code convertPlus == true} all ‘+’ chars in the decoded output are converted to ‘ ‘
(white space)
- if {@code throwOnFailure == true}, an {@link IllegalArgumentException} is thrown for
invalid inputs. Else, U+FFFd is emitted to the output in place of invalid input octets.
| UriCodec::decode | java | Reginer/aosp-android-jar | android-34/src/android/net/UriCodec.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/android/net/UriCodec.java | MIT |
public String getFormat()
{
return "PKCS#8";
} |
return the encoding format we produce in getEncoded().
@return the string "PKCS#8"
| JCEDHPrivateKey::getFormat | java | Reginer/aosp-android-jar | android-35/src/com/android/org/bouncycastle/jce/provider/JCEDHPrivateKey.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/org/bouncycastle/jce/provider/JCEDHPrivateKey.java | MIT |
public byte[] getEncoded()
{
try
{
if (info != null)
{
return info.getEncoded(ASN1Encoding.DER);
}
PrivateKeyInfo info = new PrivateKeyInfo(new AlgorithmIdentifier(PKCSObjectIdentifiers.dhKeyAgreement, new DHParameter(dhSpec.getP(), dhSpec.getG(), dhSpec.getL())), new ASN1Integer(getX()));
return info.getEncoded(ASN1Encoding.DER);
}
catch (IOException e)
{
return null;
}
} |
Return a PKCS8 representation of the key. The sequence returned
represents a full PrivateKeyInfo object.
@return a PKCS8 representation of the key.
| JCEDHPrivateKey::getEncoded | java | Reginer/aosp-android-jar | android-35/src/com/android/org/bouncycastle/jce/provider/JCEDHPrivateKey.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/org/bouncycastle/jce/provider/JCEDHPrivateKey.java | MIT |
static X500Name getEncodedIssuerPrincipal(Object cert)
{
if (cert instanceof X509Certificate)
{
return getIssuerPrincipal((X509Certificate)cert);
}
else
{
return getX500Name((X500Principal)((X509AttributeCertificate)cert).getIssuer().getPrincipals()[0]);
}
} |
Returns the issuer of an attribute certificate or certificate.
@param cert The attribute certificate or certificate.
@return The issuer as <code>X500Principal</code>.
| PrincipalUtils::getEncodedIssuerPrincipal | java | Reginer/aosp-android-jar | android-35/src/com/android/org/bouncycastle/jce/provider/PrincipalUtils.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/com/android/org/bouncycastle/jce/provider/PrincipalUtils.java | MIT |
public void setUseBackground(boolean useBackground) {
mUseBackground = useBackground;
updateColorAndBackgroundVisibility();
} |
Whether or not to render the lock icon background. Mainly used for UDPFS.
| LockIconView::setUseBackground | java | Reginer/aosp-android-jar | android-32/src/com/android/keyguard/LockIconView.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/keyguard/LockIconView.java | MIT |
public void clearIcon() {
updateIcon(ICON_NONE, false);
} |
Updates the icon its default state where no visual is shown.
| LockIconView::clearIcon | java | Reginer/aosp-android-jar | android-32/src/com/android/keyguard/LockIconView.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/keyguard/LockIconView.java | MIT |
public void updateIcon(@IconType int icon, boolean aod) {
mIconType = icon;
mAod = aod;
mLockIcon.setImageState(getLockIconState(mIconType, mAod), true);
} |
Transition the current icon to a new state
@param icon type (ie: lock icon, unlock icon, fingerprint icon)
@param aod whether to use the aod icon variant (some icons don't have aod variants and will
therefore show no icon)
| LockIconView::updateIcon | java | Reginer/aosp-android-jar | android-32/src/com/android/keyguard/LockIconView.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/keyguard/LockIconView.java | MIT |
default LongPredicate and(LongPredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) && other.test(value);
} |
Returns a composed predicate that represents a short-circuiting logical
AND of this predicate and another. When evaluating the composed
predicate, if this predicate is {@code false}, then the {@code other}
predicate is not evaluated.
<p>Any exceptions thrown during evaluation of either predicate are relayed
to the caller; if evaluation of this predicate throws an exception, the
{@code other} predicate will not be evaluated.
@param other a predicate that will be logically-ANDed with this
predicate
@return a composed predicate that represents the short-circuiting logical
AND of this predicate and the {@code other} predicate
@throws NullPointerException if other is null
| and | java | Reginer/aosp-android-jar | android-35/src/java/util/function/LongPredicate.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/java/util/function/LongPredicate.java | MIT |
default LongPredicate negate() {
return (value) -> !test(value);
} |
Returns a predicate that represents the logical negation of this
predicate.
@return a predicate that represents the logical negation of this
predicate
| negate | java | Reginer/aosp-android-jar | android-35/src/java/util/function/LongPredicate.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/java/util/function/LongPredicate.java | MIT |
default LongPredicate or(LongPredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) || other.test(value);
} |
Returns a composed predicate that represents a short-circuiting logical
OR of this predicate and another. When evaluating the composed
predicate, if this predicate is {@code true}, then the {@code other}
predicate is not evaluated.
<p>Any exceptions thrown during evaluation of either predicate are relayed
to the caller; if evaluation of this predicate throws an exception, the
{@code other} predicate will not be evaluated.
@param other a predicate that will be logically-ORed with this
predicate
@return a composed predicate that represents the short-circuiting logical
OR of this predicate and the {@code other} predicate
@throws NullPointerException if other is null
| or | java | Reginer/aosp-android-jar | android-35/src/java/util/function/LongPredicate.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-35/src/java/util/function/LongPredicate.java | MIT |
public static FieldClassificationEventLogger createLogger() {
return new FieldClassificationEventLogger();
} |
A factory constructor to create FieldClassificationEventLogger.
| FieldClassificationEventLogger::createLogger | java | Reginer/aosp-android-jar | android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | MIT |
public void startNewLogForRequest() {
if (!mEventInternal.isEmpty()) {
Slog.w(TAG, "FieldClassificationEventLogger is not empty before starting for a new "
+ "request");
}
mEventInternal = Optional.of(new FieldClassificationEventInternal());
} |
Reset mEventInternal before logging for a new request. It shall be called for each
FieldClassification request.
| FieldClassificationEventLogger::startNewLogForRequest | java | Reginer/aosp-android-jar | android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | MIT |
public void maybeSetLatencyMillis(long timestamp) {
mEventInternal.ifPresent(event -> {
event.mLatencyClassificationRequestMillis = timestamp;
});
} |
Set latency as long as mEventInternal presents.
| FieldClassificationEventLogger::maybeSetLatencyMillis | java | Reginer/aosp-android-jar | android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | MIT |
public void logAndEndEvent() {
if (!mEventInternal.isPresent()) {
Slog.w(TAG, "Shouldn't be logging AutofillFieldClassificationEventInternal again for "
+ "same event");
return;
}
FieldClassificationEventInternal event = mEventInternal.get();
if (sVerbose) {
Slog.v(TAG, "Log AutofillFieldClassificationEventReported:"
+ " mLatencyClassificationRequestMillis="
+ event.mLatencyClassificationRequestMillis);
}
FrameworkStatsLog.write(
AUTOFILL_FIELD_CLASSIFICATION_EVENT_REPORTED,
event.mLatencyClassificationRequestMillis);
mEventInternal = Optional.empty();
} |
Log an AUTOFILL_FIELD_CLASSIFICATION_EVENT_REPORTED event.
| FieldClassificationEventLogger::logAndEndEvent | java | Reginer/aosp-android-jar | android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/com/android/server/autofill/FieldClassificationEventLogger.java | MIT |
public void stop() {
mIsStopping = true;
sendMessage(obtainMessage(BackupHandler.MSG_STOP));
} |
Put the BackupHandler into a stopping state where the remaining messages on the queue will be
silently dropped and the {@link WakeLock} held by the {@link UserBackupManagerService} will
then be released.
| BackupHandler::stop | java | Reginer/aosp-android-jar | android-32/src/com/android/server/backup/internal/BackupHandler.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/backup/internal/BackupHandler.java | MIT |
private void logTuneStateChanged(int state, SessionState sessionState,
@Nullable TvInputState inputState) {
int tisUid = Process.INVALID_UID;
int inputType = FrameworkStatsLog.TIF_TUNE_STATE_CHANGED__TYPE__TIF_INPUT_TYPE_UNKNOWN;
int inputId = 0;
int hdmiPort = 0;
if (inputState != null) {
tisUid = inputState.uid;
inputType = inputState.info.getType();
if (inputType == TvInputInfo.TYPE_TUNER) {
inputType = FrameworkStatsLog.TIF_TUNE_STATE_CHANGED__TYPE__TUNER;
}
inputId = inputState.inputNumber;
HdmiDeviceInfo hdmiDeviceInfo = inputState.info.getHdmiDeviceInfo();
if (hdmiDeviceInfo != null) {
hdmiPort = hdmiDeviceInfo.getPortId();
}
}
FrameworkStatsLog.write(FrameworkStatsLog.TIF_TUNE_CHANGED,
new int[]{sessionState.callingUid,
tisUid},
new String[]{"tif_player", "tv_input_service"},
state,
sessionState.sessionId,
inputType,
inputId,
hdmiPort);
} |
Log Tune state changes to {@link FrameworkStatsLog}.
<p><b>WARNING</b> Any changes to this field should be carefully reviewed for privacy.
Inspect the code at:
<ul>
<li>framework/base/cmds/statsd/src/atoms.proto#TifTuneState
<li>{@link #logTuneStateChanged}
<li>{@link TvInputManagerService.BinderService#createSession}
<li>{@link SessionState#sessionId}
</ul>
| BinderService::logTuneStateChanged | java | Reginer/aosp-android-jar | android-33/src/com/android/server/tv/TvInputManagerService.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/com/android/server/tv/TvInputManagerService.java | MIT |
public hc_nodeinsertbeforerefchildnull(final DOMTestDocumentBuilderFactory factory) throws org.w3c.domts.DOMTestIncompatibleException {
super(factory);
//
// check if loaded documents are supported for content type
//
String contentType = getContentType();
preload(contentType, "hc_staff", true);
} |
Constructor.
@param factory document factory, may not be null
@throws org.w3c.domts.DOMTestIncompatibleException Thrown if test is not compatible with parser configuration
| hc_nodeinsertbeforerefchildnull::hc_nodeinsertbeforerefchildnull | java | Reginer/aosp-android-jar | android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | MIT |
public void runTest() throws Throwable {
Document doc;
NodeList elementList;
Node employeeNode;
NodeList childList;
Node refChild = null;
Node newChild;
Node child;
String childName;
Node insertedNode;
doc = (Document) load("hc_staff", true);
elementList = doc.getElementsByTagName("p");
employeeNode = elementList.item(1);
childList = employeeNode.getChildNodes();
newChild = doc.createElement("br");
insertedNode = employeeNode.insertBefore(newChild, refChild);
child = employeeNode.getLastChild();
childName = child.getNodeName();
assertEqualsAutoCase("element", "nodeName", "br", childName);
} |
Runs the test case.
@throws Throwable Any uncaught exception causes test to fail
| hc_nodeinsertbeforerefchildnull::runTest | java | Reginer/aosp-android-jar | android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | MIT |
public String getTargetURI() {
return "http://www.w3.org/2001/DOM-Test-Suite/level1/core/hc_nodeinsertbeforerefchildnull";
} |
Gets URI that identifies the test.
@return uri identifier of test
| hc_nodeinsertbeforerefchildnull::getTargetURI | java | Reginer/aosp-android-jar | android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | MIT |
public static void main(final String[] args) {
DOMTestCase.doMain(hc_nodeinsertbeforerefchildnull.class, args);
} |
Runs this test from the command line.
@param args command line arguments
| hc_nodeinsertbeforerefchildnull::main | java | Reginer/aosp-android-jar | android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-34/src/org/w3c/domts/level1/core/hc_nodeinsertbeforerefchildnull.java | MIT |
public documentimportnode21(final DOMTestDocumentBuilderFactory factory) throws org.w3c.domts.DOMTestIncompatibleException {
org.w3c.domts.DocumentBuilderSetting[] settings =
new org.w3c.domts.DocumentBuilderSetting[] {
org.w3c.domts.DocumentBuilderSetting.namespaceAware,
org.w3c.domts.DocumentBuilderSetting.notExpandEntityReferences
};
DOMTestDocumentBuilderFactory testFactory = factory.newInstance(settings);
setFactory(testFactory);
//
// check if loaded documents are supported for content type
//
String contentType = getContentType();
preload(contentType, "staffNS", true);
} |
Constructor.
@param factory document factory, may not be null
@throws org.w3c.domts.DOMTestIncompatibleException Thrown if test is not compatible with parser configuration
| documentimportnode21::documentimportnode21 | java | Reginer/aosp-android-jar | android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | MIT |
public void runTest() throws Throwable {
Document doc;
DocumentType docTypeNull = null;
Document docImp;
DOMImplementation domImpl;
NodeList addressList;
NodeList addressChildList;
Element element;
EntityReference entRef2;
EntityReference entRefImp2;
EntityReference entRef3;
EntityReference entRefImp3;
String nodeName2;
String nodeName3;
String nodeNameImp2;
String nodeNameImp3;
NodeList nodes;
Node nodeImp3;
Node nodeImp2;
String nodeValueImp2;
String nodeValueImp3;
doc = (Document) load("staffNS", true);
domImpl = doc.getImplementation();
docImp = domImpl.createDocument("http://www.w3.org/DOM/Test", "a:b", docTypeNull);
addressList = doc.getElementsByTagName("address");
element = (Element) addressList.item(1);
addressChildList = element.getChildNodes();
entRef2 = (EntityReference) addressChildList.item(0);
entRef3 = (EntityReference) addressChildList.item(2);
entRefImp2 = (EntityReference) docImp.importNode(entRef2, true);
entRefImp3 = (EntityReference) docImp.importNode(entRef3, false);
nodeName2 = entRef2.getNodeName();
nodeName3 = entRef3.getNodeName();
nodeNameImp2 = entRefImp2.getNodeName();
nodeNameImp3 = entRefImp3.getNodeName();
assertEquals("documentimportnode21_Ent2NodeName", nodeName2, nodeNameImp2);
assertEquals("documentimportnode21_Ent3NodeName", nodeName3, nodeNameImp3);
entRefImp2 = (EntityReference) doc.importNode(entRef2, true);
entRefImp3 = (EntityReference) doc.importNode(entRef3, false);
nodes = entRefImp2.getChildNodes();
nodeImp2 = nodes.item(0);
nodeValueImp2 = nodeImp2.getNodeValue();
nodes = entRefImp3.getChildNodes();
nodeImp3 = nodes.item(0);
nodeValueImp3 = nodeImp3.getNodeValue();
assertEquals("documentimportnode21_Ent2NodeValue", "1900 Dallas Road", nodeValueImp2);
assertEquals("documentimportnode21_Ent3Nodevalue", "Texas", nodeValueImp3);
} |
Runs the test case.
@throws Throwable Any uncaught exception causes test to fail
| documentimportnode21::runTest | java | Reginer/aosp-android-jar | android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | MIT |
public String getTargetURI() {
return "http://www.w3.org/2001/DOM-Test-Suite/level2/core/documentimportnode21";
} |
Gets URI that identifies the test.
@return uri identifier of test
| documentimportnode21::getTargetURI | java | Reginer/aosp-android-jar | android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | MIT |
public static void main(final String[] args) {
DOMTestCase.doMain(documentimportnode21.class, args);
} |
Runs this test from the command line.
@param args command line arguments
| documentimportnode21::main | java | Reginer/aosp-android-jar | android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-33/src/org/w3c/domts/level2/core/documentimportnode21.java | MIT |
public prefix02(final DOMTestDocumentBuilderFactory factory) throws org.w3c.domts.DOMTestIncompatibleException {
super(factory);
//
// check if loaded documents are supported for content type
//
String contentType = getContentType();
preload(contentType, "staffNS", false);
} |
Constructor.
@param factory document factory, may not be null
@throws org.w3c.domts.DOMTestIncompatibleException Thrown if test is not compatible with parser configuration
| prefix02::prefix02 | java | Reginer/aosp-android-jar | android-32/src/org/w3c/domts/level2/core/prefix02.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/org/w3c/domts/level2/core/prefix02.java | MIT |
public void runTest() throws Throwable {
Document doc;
NodeList elementList;
Node testEmployee;
Node textNode;
String prefix;
doc = (Document) load("staffNS", false);
elementList = doc.getElementsByTagName("emp:employeeId");
testEmployee = elementList.item(0);
assertNotNull("empEmployeeNotNull", testEmployee);
textNode = testEmployee.getFirstChild();
prefix = textNode.getPrefix();
assertNull("textNodePrefix", prefix);
} |
Runs the test case.
@throws Throwable Any uncaught exception causes test to fail
| prefix02::runTest | java | Reginer/aosp-android-jar | android-32/src/org/w3c/domts/level2/core/prefix02.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/org/w3c/domts/level2/core/prefix02.java | MIT |
public String getTargetURI() {
return "http://www.w3.org/2001/DOM-Test-Suite/level2/core/prefix02";
} |
Gets URI that identifies the test.
@return uri identifier of test
| prefix02::getTargetURI | java | Reginer/aosp-android-jar | android-32/src/org/w3c/domts/level2/core/prefix02.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/org/w3c/domts/level2/core/prefix02.java | MIT |
public static void main(final String[] args) {
DOMTestCase.doMain(prefix02.class, args);
} |
Runs this test from the command line.
@param args command line arguments
| prefix02::main | java | Reginer/aosp-android-jar | android-32/src/org/w3c/domts/level2/core/prefix02.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/org/w3c/domts/level2/core/prefix02.java | MIT |
private UinputBridge(IBinder token, long ptr) {
mPtr = ptr;
mToken = token;
mCloseGuard.open("close");
} |
Send an axis value.
Available axes are:
<li> Left joystick: AXIS_X, AXIS_Y
<li> Right joystick: AXIS_Z, AXIS_RZ
<li> Analog triggers: AXIS_LTRIGGER, AXIS_RTRIGGER
<li> DPad: AXIS_HAT_X, AXIS_HAT_Y
@param axis is a MotionEvent.AXIS_* value.
@param value is a value between -1 and 1 (inclusive)
private static native void nativeSendGamepadAxisValue(long ptr, int axis, float value);
public UinputBridge(IBinder token, String name, int width, int height, int maxPointers)
throws IOException {
if (width < 1 || height < 1) {
throw new IllegalArgumentException("Touchpad must be at least 1x1.");
}
if (maxPointers < 1 || maxPointers > 32) {
throw new IllegalArgumentException("Touchpad must support between 1 and 32 pointers.");
}
if (token == null) {
throw new IllegalArgumentException("Token cannot be null");
}
mPtr = nativeOpen(name, token.toString(), width, height, maxPointers);
if (mPtr == 0) {
throw new IOException("Could not open uinput device " + name);
}
mToken = token;
mCloseGuard.open("close");
}
/** Constructor used by static factory methods | UinputBridge::UinputBridge | java | Reginer/aosp-android-jar | android-32/src/com/android/server/tv/UinputBridge.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/tv/UinputBridge.java | MIT |
public static UinputBridge openGamepad(IBinder token, String name)
throws IOException {
if (token == null) {
throw new IllegalArgumentException("Token cannot be null");
}
long ptr = nativeGamepadOpen(name, token.toString());
if (ptr == 0) {
throw new IOException("Could not open uinput device " + name);
}
return new UinputBridge(token, ptr);
} |
Send an axis value.
Available axes are:
<li> Left joystick: AXIS_X, AXIS_Y
<li> Right joystick: AXIS_Z, AXIS_RZ
<li> Analog triggers: AXIS_LTRIGGER, AXIS_RTRIGGER
<li> DPad: AXIS_HAT_X, AXIS_HAT_Y
@param axis is a MotionEvent.AXIS_* value.
@param value is a value between -1 and 1 (inclusive)
private static native void nativeSendGamepadAxisValue(long ptr, int axis, float value);
public UinputBridge(IBinder token, String name, int width, int height, int maxPointers)
throws IOException {
if (width < 1 || height < 1) {
throw new IllegalArgumentException("Touchpad must be at least 1x1.");
}
if (maxPointers < 1 || maxPointers > 32) {
throw new IllegalArgumentException("Touchpad must support between 1 and 32 pointers.");
}
if (token == null) {
throw new IllegalArgumentException("Token cannot be null");
}
mPtr = nativeOpen(name, token.toString(), width, height, maxPointers);
if (mPtr == 0) {
throw new IOException("Could not open uinput device " + name);
}
mToken = token;
mCloseGuard.open("close");
}
/** Constructor used by static factory methods
private UinputBridge(IBinder token, long ptr) {
mPtr = ptr;
mToken = token;
mCloseGuard.open("close");
}
/** Opens a UinputBridge that supports gamepad buttons and axes. | UinputBridge::openGamepad | java | Reginer/aosp-android-jar | android-32/src/com/android/server/tv/UinputBridge.java | https://github.com/Reginer/aosp-android-jar/blob/master/android-32/src/com/android/server/tv/UinputBridge.java | MIT |
This dataset contains Java functions with their documentation comments extracted from GitHub repositories.
code
: The Java function codedocstring
: Documentation comment for the functionfunc_name
: Function namelanguage
: Programming language (always "Java")repo
: Source repository namepath
: File path within the repositoryurl
: GitHub URL to the source filelicense
: License of the source codeThe dataset contains the following splits:
from datasets import load_dataset
# Load the dataset
dataset = load_dataset("Shuu12121/java-codesearch-dataset-open")
# Access the training split
train_data = dataset["train"]
# Example: Print the first sample
print(train_data[0])
This dataset was created by scraping Java code from GitHub repositories. Each function includes its documentation comment and license information.
This dataset contains code from various repositories with different licenses. Each sample includes its original license information in the license
field.